Hello, Anymal
Hello, Anymal
Anymal (short for "any animal") makes animal economies programmable,
unlocking opportunities for developers to plug in and expand within trillion-dollar animal care industries.
Anymal Protocol makes any animal economy programmable, enabling developers to tap into and expand vast multi-trillion-dollar ecosystems—from pet care to wildlife.
Anymal (short for "any animal") makes animal economies programmable, unlocking opportunities for developers to plug in and expand within trillion-dollar animal care industries.
👋
👋
✨
✨
✨
Build
Build
Build
Plug-in Network
Plug-in Network
Anymal’s plug-in architecture enables integration and innovation across established animal economies, offering developers instant scale.
Plug-in Network
Anymal’s plug-in architecture enables integration and innovation across established animal economies, offering developers instant scale.
Plug-in Network
Anymal’s plug-in architecture enables integration and innovation across established animal economies, offering developers instant scale.
You 🐾
import React, { useState } from 'react';
import { createAnymal, addToAnymalNetwork }
from 'anymal-protocol-js';
const CreateAnymal = () => {
const [species, setSpecies] = useState('');
const [breed, setBreed] = useState('');
const [age, setAge] = useState('');
const [gender, setGender] = useState('');
const [photoUrl, setPhotoUrl] = useState('');
const handleCreateAndAddAnymal = async () => {
try {
const ownerId = 'alice.testnet';
const createdAnymal = await createAnymal({
species,
breed,
age: parseInt(age, 10),
gender,
photo: photoUrl,
ownerId,
});
const networkResponse = await addToAnymalNetwork({
animalId: createdAnymal.animal_id,
ownerId,
});
console.log('Anymal Added to Network:', networkResponse);
alert('Anymal created and added to the network successfully!');
} catch (error) {
console.error('Error creating and adding Anymal:', error);
alert('Failed to create and add Anymal.');
}
};
return (
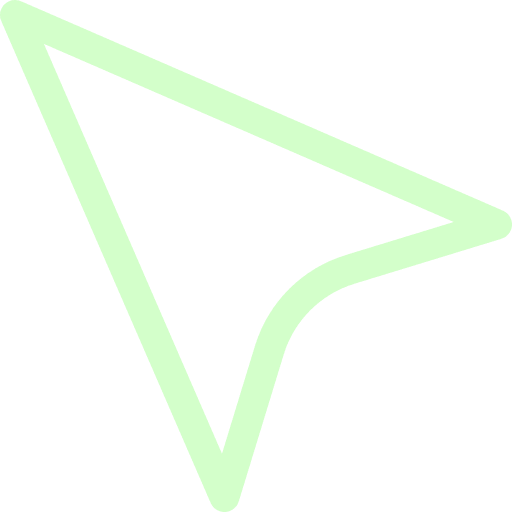
You 🐾
import React, { useState } from 'react';
import { createAnymal, addToAnymalNetwork }
from 'anymal-protocol-js';
const CreateAnymal = () => {
const [species, setSpecies] = useState('');
const [breed, setBreed] = useState('');
const [age, setAge] = useState('');
const [gender, setGender] = useState('');
const [photoUrl, setPhotoUrl] = useState('');
const handleCreateAndAddAnymal = async () => {
try {
const ownerId = 'alice.testnet';
const createdAnymal = await createAnymal({
species,
breed,
age: parseInt(age, 10),
gender,
photo: photoUrl,
ownerId,
});
const networkResponse = await addToAnymalNetwork({
animalId: createdAnymal.animal_id,
ownerId,
});
console.log('Anymal Added to Network:', networkResponse);
alert('Anymal created and added to the network successfully!');
} catch (error) {
console.error('Error creating and adding Anymal:', error);
alert('Failed to create and add Anymal.');
}
};
return (
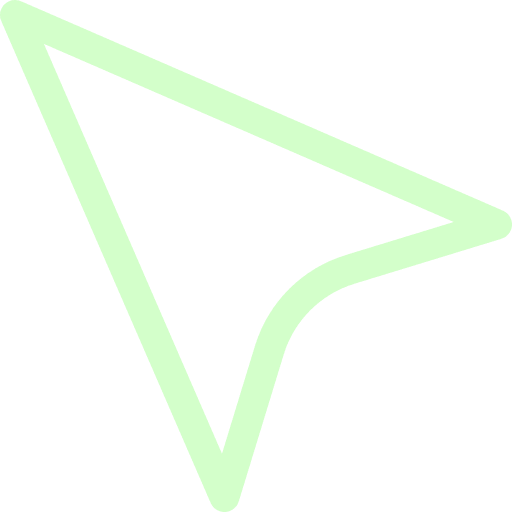
Anymal IDx
Programmable IDs serve as modular building blocks, fostering adaptable, scalable applications tailored to diverse animal needs.
You 🐾
import React, { useState } from 'react';
import { createAnymal, addToAnymalNetwork }
from 'anymal-protocol-js';
const CreateAnymal = () => {
const [species, setSpecies] = useState('');
const [breed, setBreed] = useState('');
const [age, setAge] = useState('');
const [gender, setGender] = useState('');
const [photoUrl, setPhotoUrl] = useState('');
const handleCreateAndAddAnymal = async () => {
try {
const ownerId = 'alice.testnet';
const createdAnymal = await createAnymal({
species,
breed,
age: parseInt(age, 10),
gender,
photo: photoUrl,
ownerId,
});
const networkResponse = await addToAnymalNetwork({
animalId: createdAnymal.animal_id,
ownerId,
});
console.log('Anymal Added to Network:', networkResponse);
alert('Anymal created and added to the network successfully!');
} catch (error) {
console.error('Error creating and adding Anymal:', error);
alert('Failed to create and add Anymal.');
}
};
return (
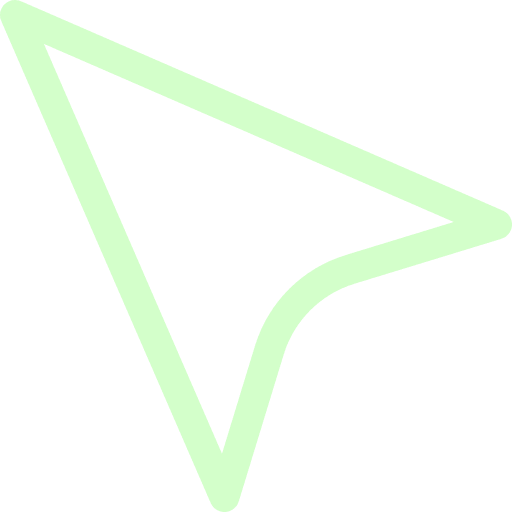
You 🐾
import React, { useState } from 'react';
import { createAnymal, addToAnymalNetwork }
from 'anymal-protocol-js';
const CreateAnymal = () => {
const [species, setSpecies] = useState('');
const [breed, setBreed] = useState('');
const [age, setAge] = useState('');
const [gender, setGender] = useState('');
const [photoUrl, setPhotoUrl] = useState('');
const handleCreateAndAddAnymal = async () => {
try {
const ownerId = 'alice.testnet';
const createdAnymal = await createAnymal({
species,
breed,
age: parseInt(age, 10),
gender,
photo: photoUrl,
ownerId,
});
const networkResponse = await addToAnymalNetwork({
animalId: createdAnymal.animal_id,
ownerId,
});
console.log('Anymal Added to Network:', networkResponse);
alert('Anymal created and added to the network successfully!');
} catch (error) {
console.error('Error creating and adding Anymal:', error);
alert('Failed to create and add Anymal.');
}
};
return (
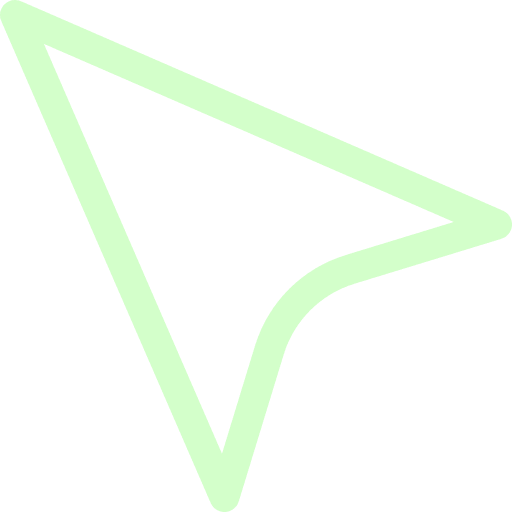
You 🐾
import React, { useState } from 'react';
import { createAnymal, addToAnymalNetwork }
from 'anymal-protocol-js';
const CreateAnymal = () => {
const [species, setSpecies] = useState('');
const [breed, setBreed] = useState('');
const [age, setAge] = useState('');
const [gender, setGender] = useState('');
const [photoUrl, setPhotoUrl] = useState('');
const handleCreateAndAddAnymal = async () => {
try {
const ownerId = 'alice.testnet';
const createdAnymal = await createAnymal({
species,
breed,
age: parseInt(age, 10),
gender,
photo: photoUrl,
ownerId,
});
const networkResponse = await addToAnymalNetwork({
animalId: createdAnymal.animal_id,
ownerId,
});
console.log('Anymal Added to Network:', networkResponse);
alert('Anymal created and added to the network successfully!');
} catch (error) {
console.error('Error creating and adding Anymal:', error);
alert('Failed to create and add Anymal.');
}
};
return (
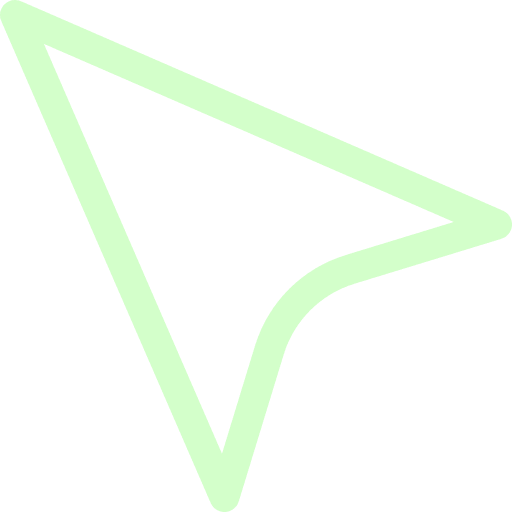
Anymal ID
Anymal ID
Programmable IDs serve as modular building blocks, fostering adaptable, scalable applications tailored to diverse animal needs.
You 🐾
import React, { useState } from 'react';
import { createAnymal, addToAnymalNetwork }
from 'anymal-protocol-js';
const CreateAnymal = () => {
const [species, setSpecies] = useState('');
const [breed, setBreed] = useState('');
const [age, setAge] = useState('');
const [gender, setGender] = useState('');
const [photoUrl, setPhotoUrl] = useState('');
const handleCreateAndAddAnymal = async () => {
try {
const ownerId = 'alice.testnet';
const createdAnymal = await createAnymal({
species,
breed,
age: parseInt(age, 10),
gender,
photo: photoUrl,
ownerId,
});
const networkResponse = await addToAnymalNetwork({
animalId: createdAnymal.animal_id,
ownerId,
});
console.log('Anymal Added to Network:', networkResponse);
alert('Anymal created and added to the network successfully!');
} catch (error) {
console.error('Error creating and adding Anymal:', error);
alert('Failed to create and add Anymal.');
}
};
return (
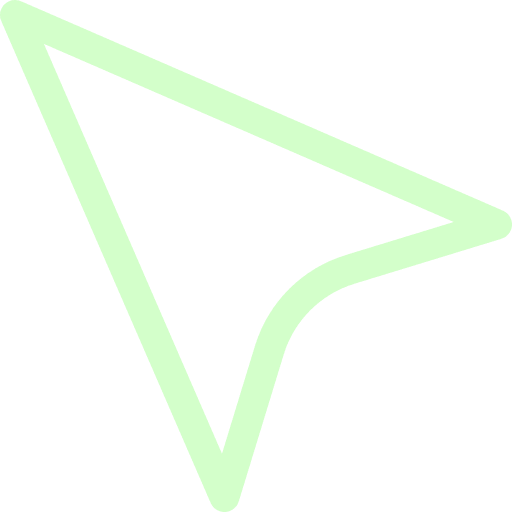
Anymal ID
Programmable IDs serve as modular building blocks, fostering adaptable, scalable applications tailored to diverse animal needs.
Livestock farming
Conservation
Wildlife management
Agricultural practices
Livestock
Veterinary services
Pharmaceutical Industry
Welfare organizations
Pet Care
Zoological parks and aquariums
Product manufacturing
Training and behavior
Livestock farming
Conservation
Wildlife management
Agricultural practices
Livestock
Veterinary services
Pharmaceutical Industry
Welfare organizations
Pet Care
Zoological parks and aquariums
Product manufacturing
Training and behavior
Livestock farming
Conservation
Wildlife management
Agricultural practices
Livestock
Veterinary services
Pharmaceutical Industry
Welfare organizations
Pet Care
Zoological parks and aquariums
Product manufacturing
Training and behavior
Ecosystem
Ecosystem
A thriving application ecosystem built on Anymal’s programmable IDs empowers flexible, customized solutions for every facet of the animal economy.
Livestock farming
Conservation
Wildlife management
Agricultural practices
Livestock
Veterinary services
Pharmaceutical Industry
Welfare organizations
Pet Care
Zoological parks and aquariums
Product manufacturing
Training and behavior
Ecosystem
A thriving application ecosystem built on Anymal’s programmable IDs empowers flexible, customized solutions for every facet of the animal economy.
Livestock farming
Conservation
Wildlife management
Agricultural practices
Livestock
Veterinary services
Pharmaceutical Industry
Welfare organizations
Pet Care
Zoological parks and aquariums
Product manufacturing
Training and behavior
Ecosystem
A thriving application ecosystem built on Anymal’s programmable IDs empowers flexible, customized solutions for every facet of the animal economy.
Ecosystem
Ecosystem
APPS
Dapps
Marketplace

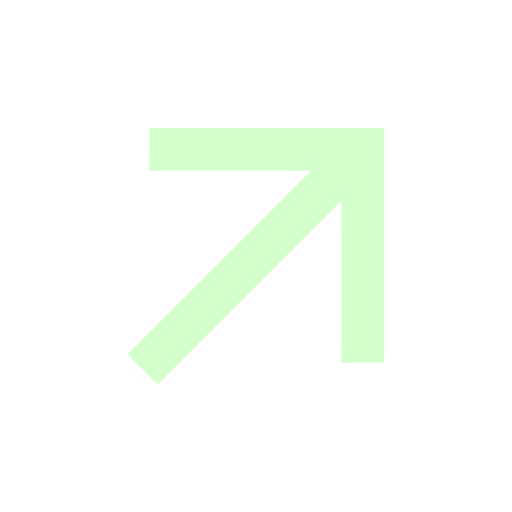
Pet Insurance

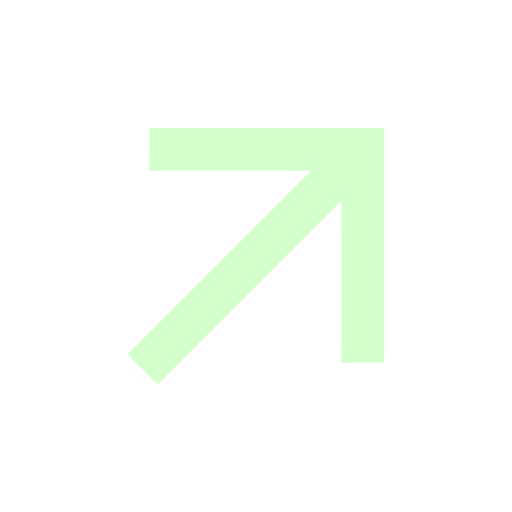
Pet Search
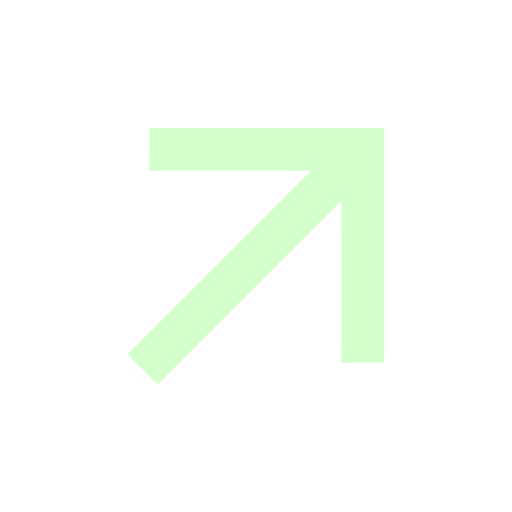
Pet Altruism Fund

Paw.fund
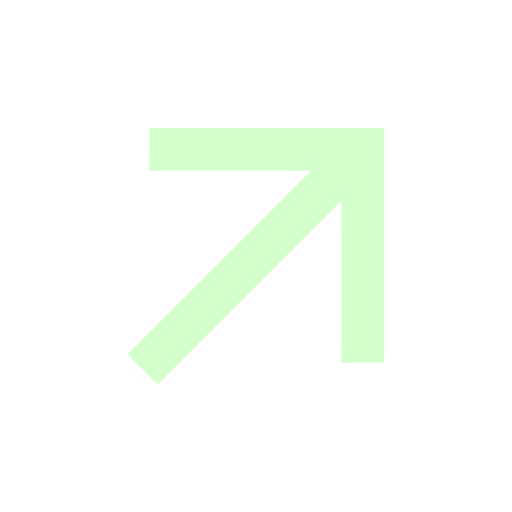
Marketplace

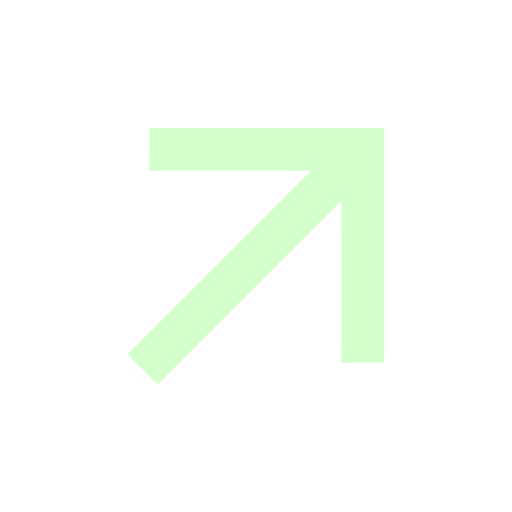
Pet Insurance

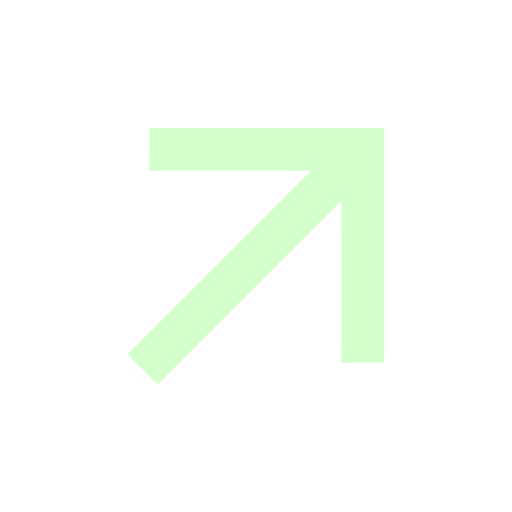
Pet Search
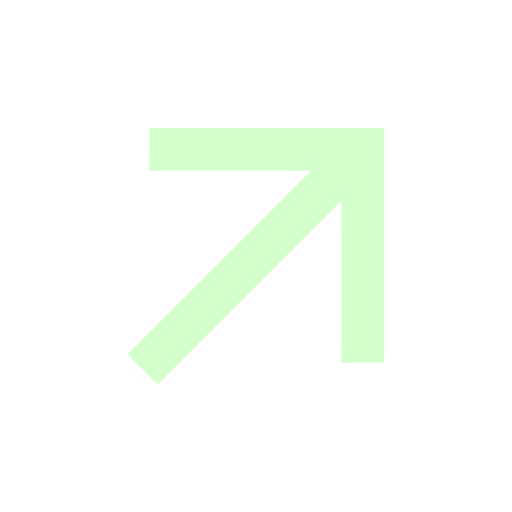
Pet Altruism Fund

Paw.fund
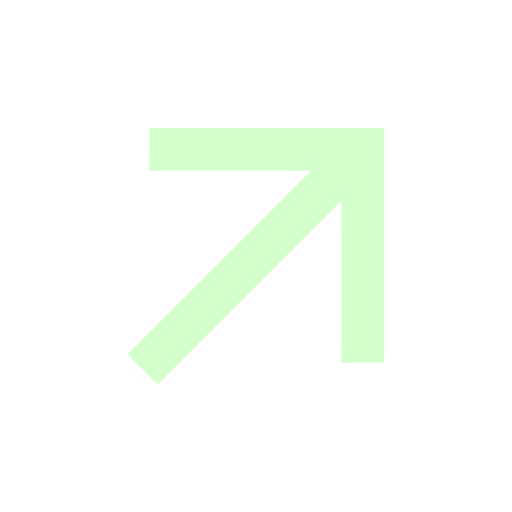
Marketplace

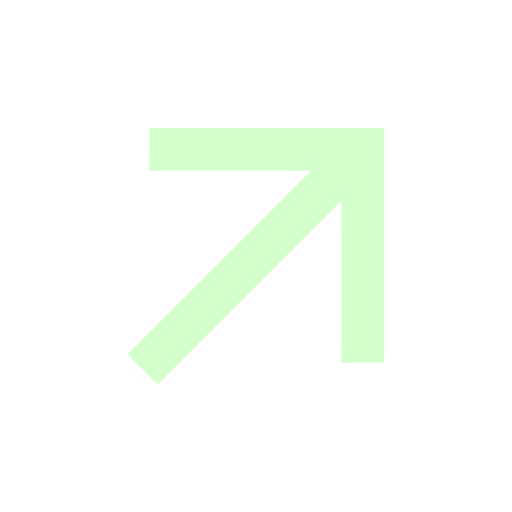
Pet Insurance

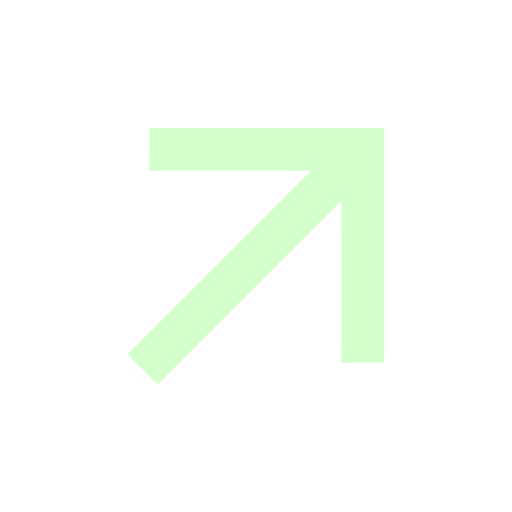
Pet Search
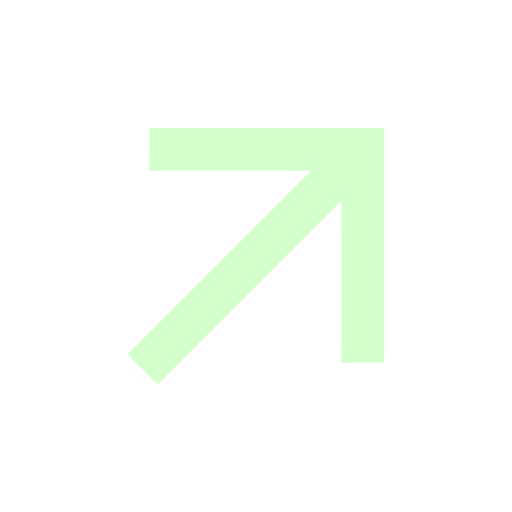
Pet Altruism Fund

Paw.fund
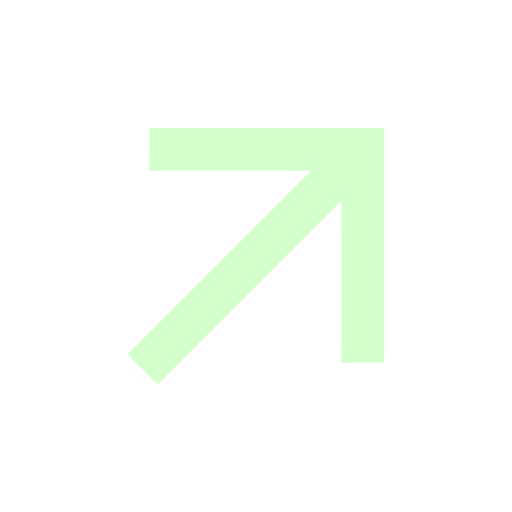
Marketplace

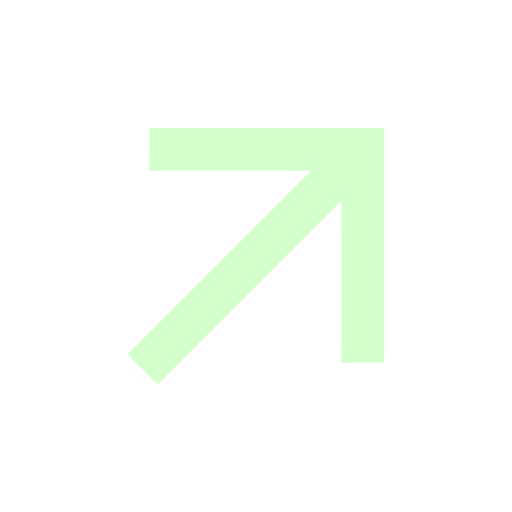
Pet Insurance

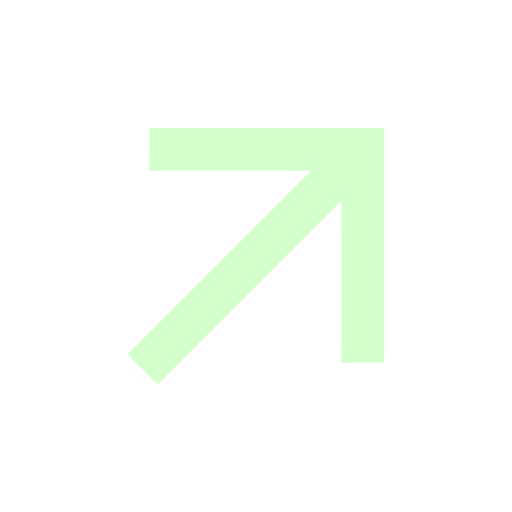
Pet Search
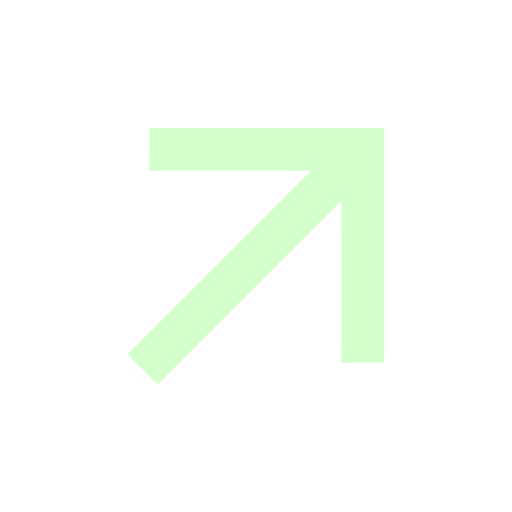
Pet Altruism Fund

Paw.fund
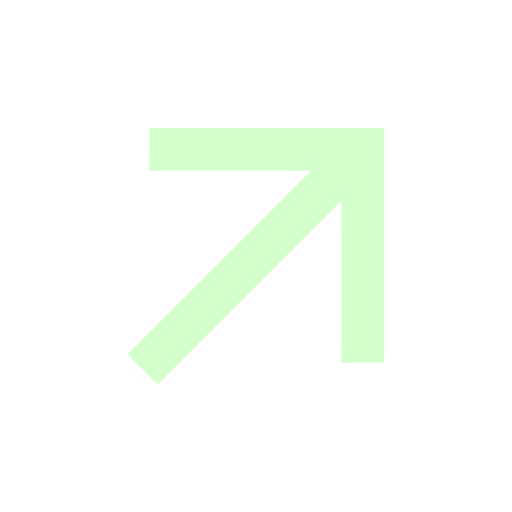
Marketplace

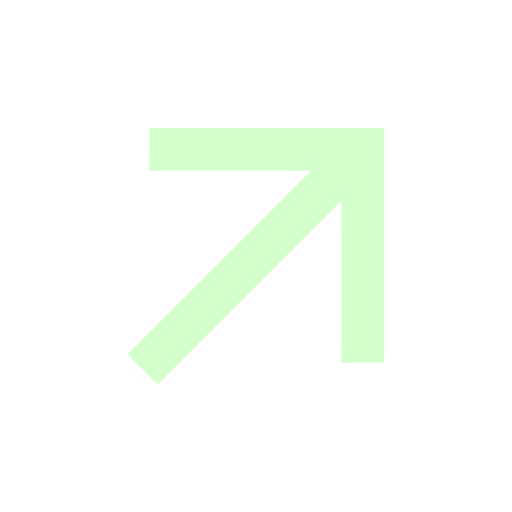
Pet Insurance

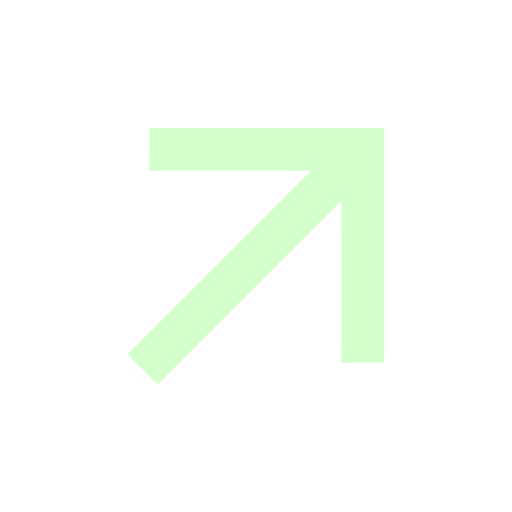
Pet Search
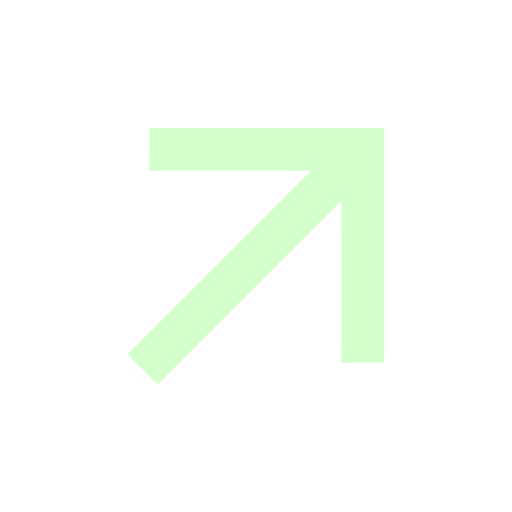
Pet Altruism Fund

Paw.fund
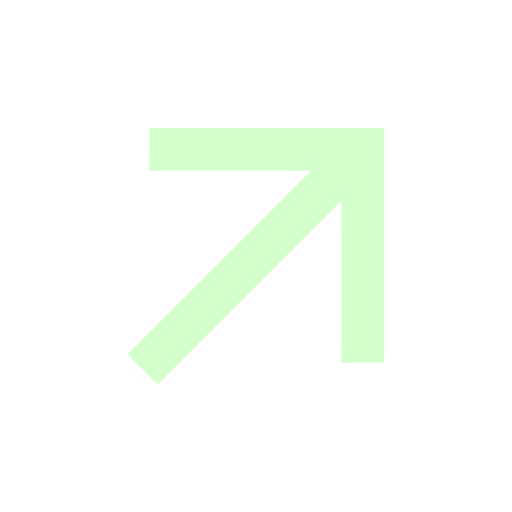
INFRASTRUCTURE
Infrastructure
Data Verification

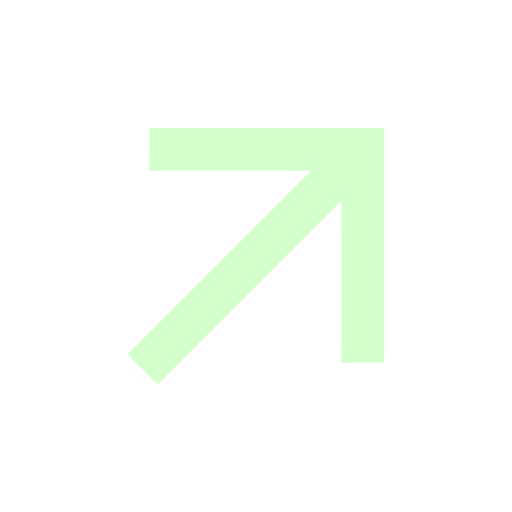
Decentralized AI

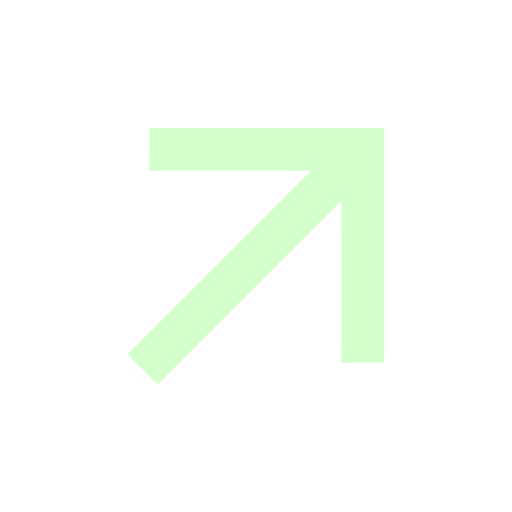
Decentralized Data

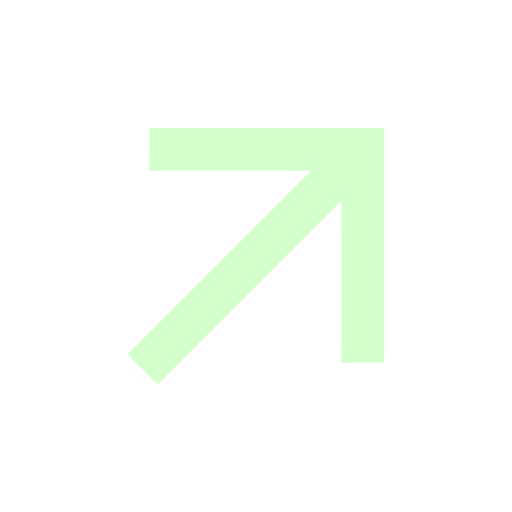
Proof of Location
Proximum
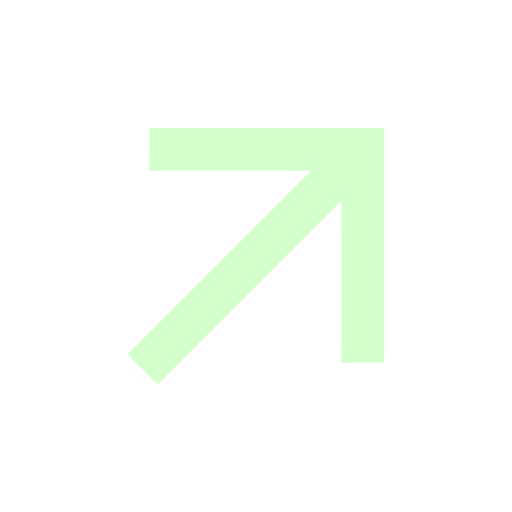
Data Verification

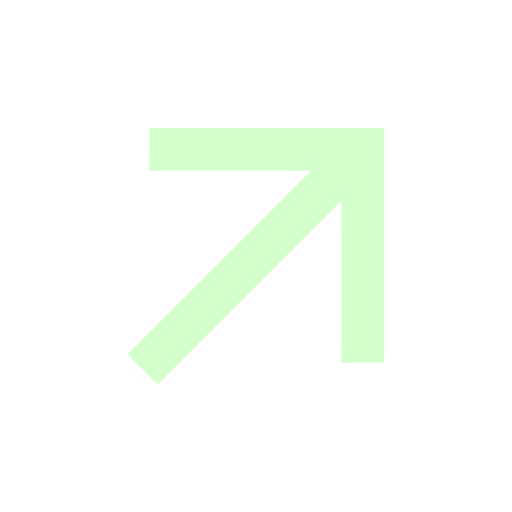
Decentralized AI

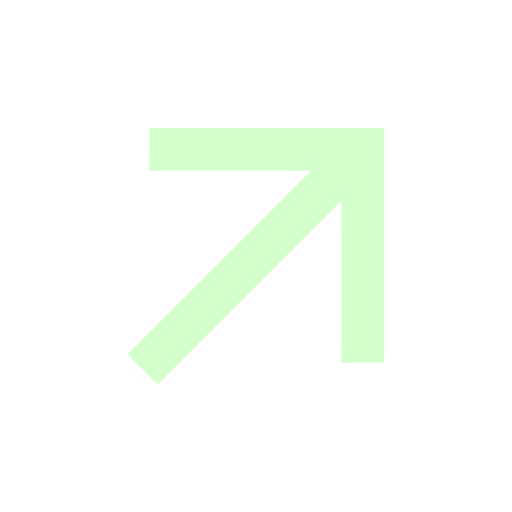
Decentralized Data

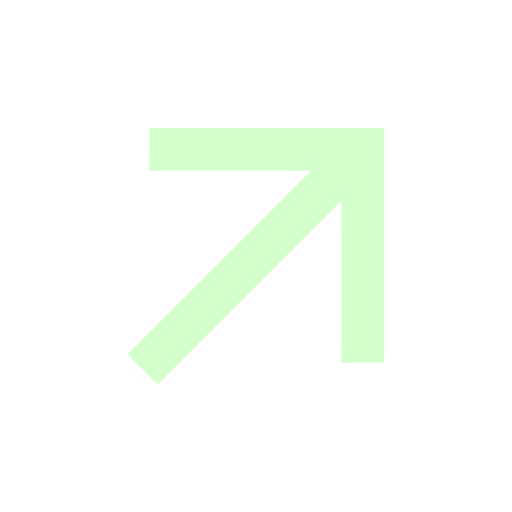
Proof of Location
Proximum
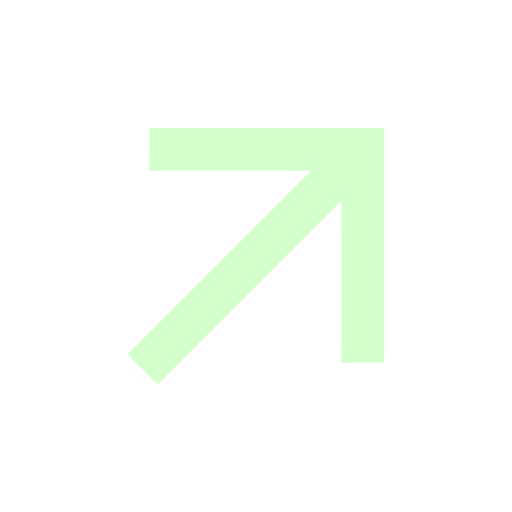
Data Verification

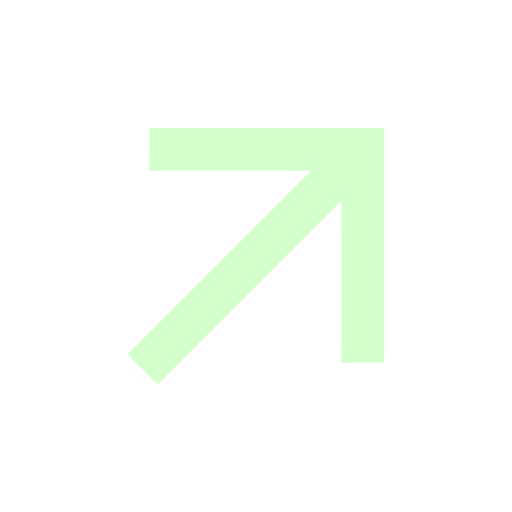
Decentralized AI

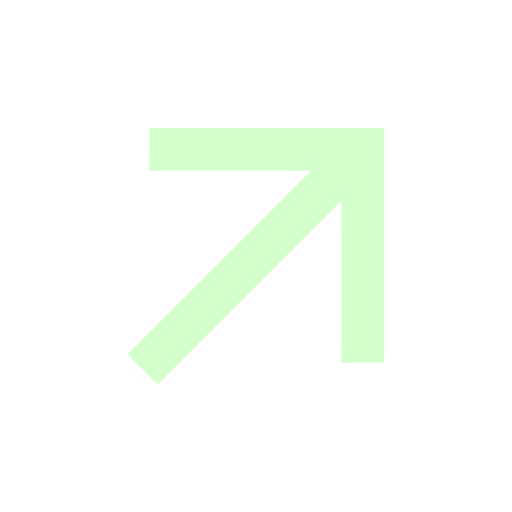
Decentralized Data

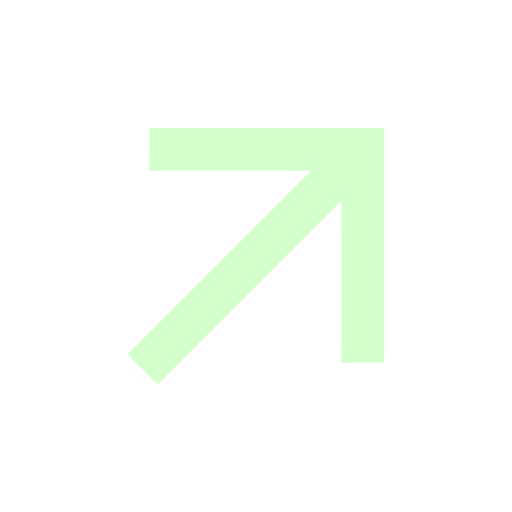
Proof of Location
Proximum
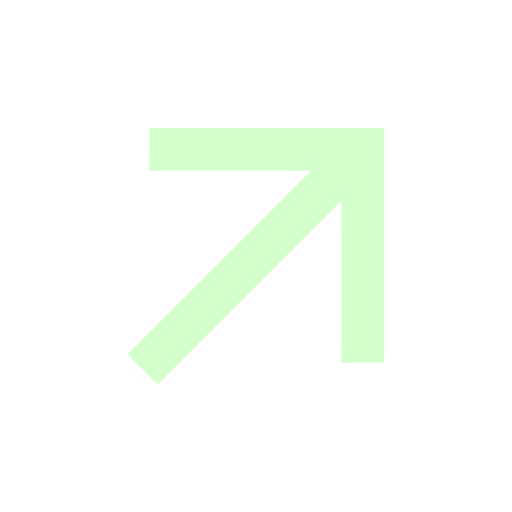
Data Verification

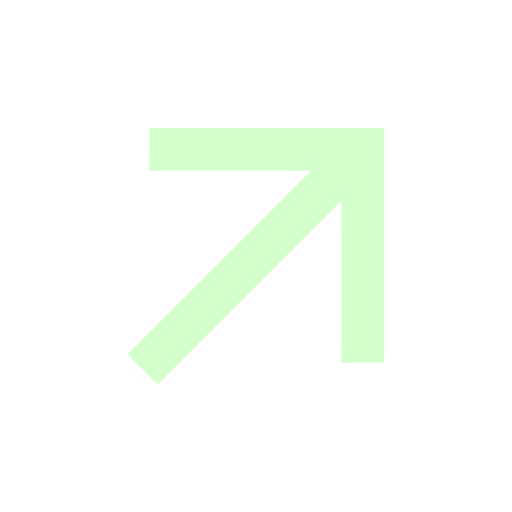
Decentralized AI

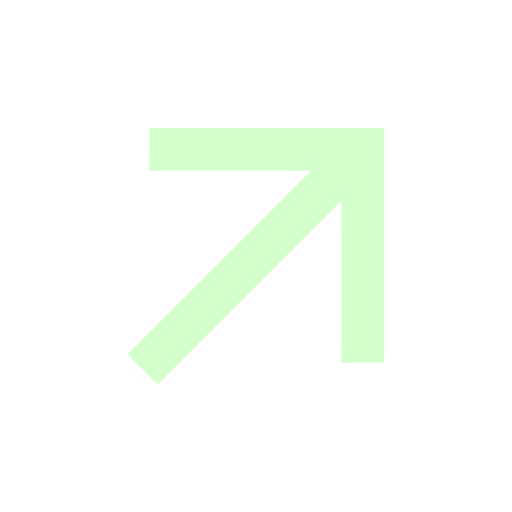
Decentralized Data

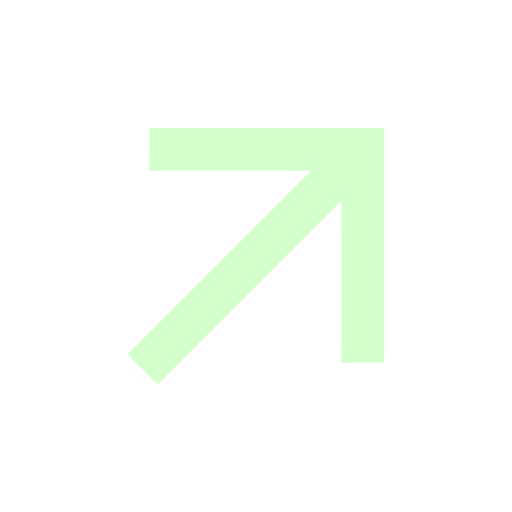
Proof of Location
Proximum
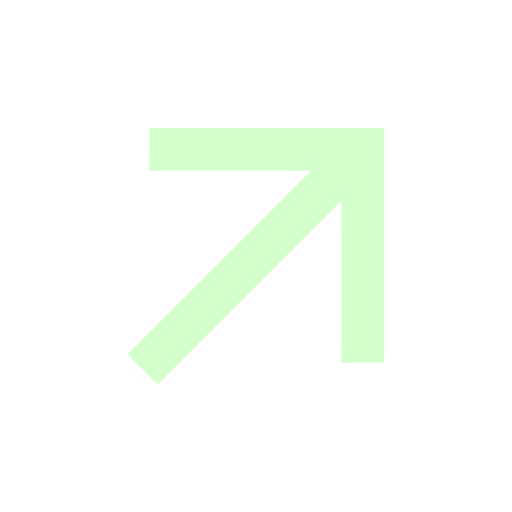
Data Verification

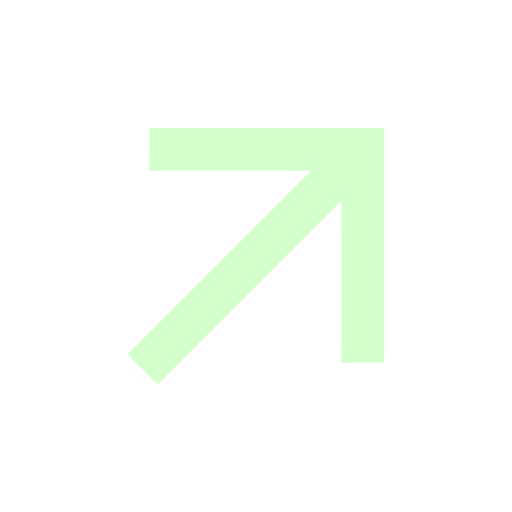
Decentralized AI

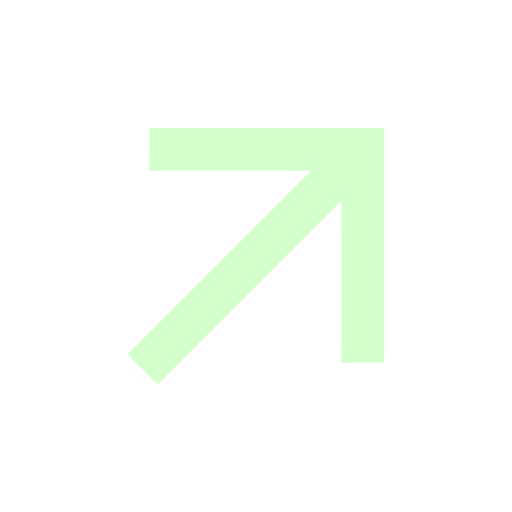
Decentralized Data

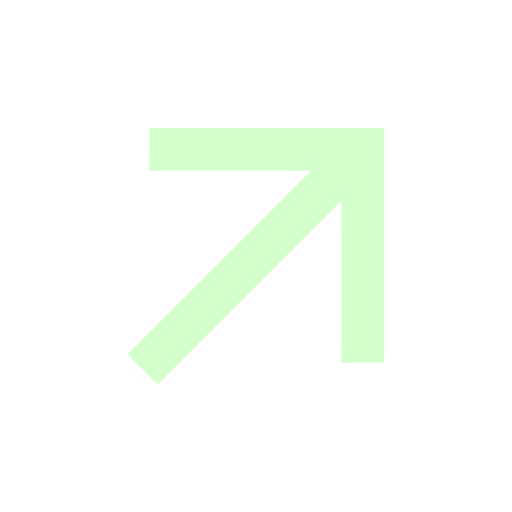
Proof of Location
Proximum
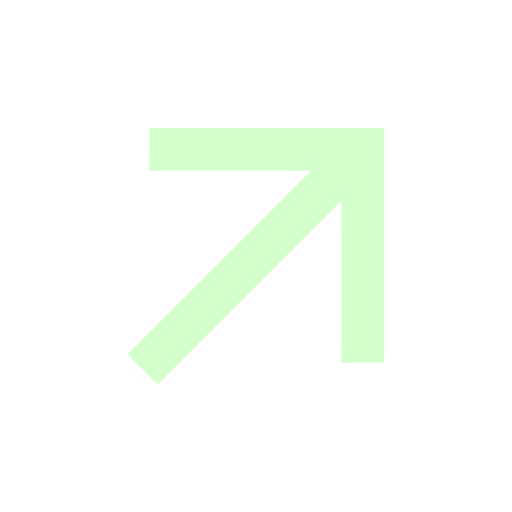
FAQ
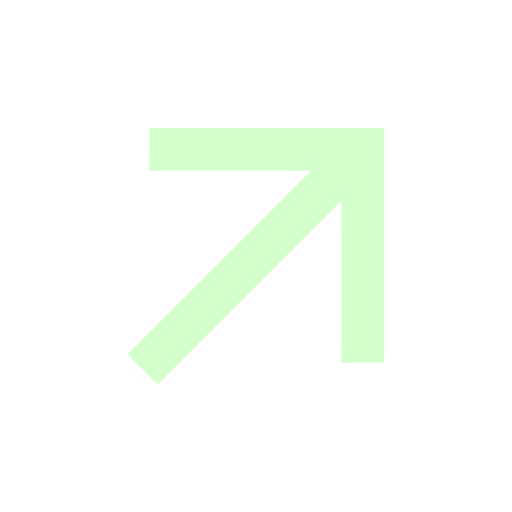
What is Anymal Protocol?
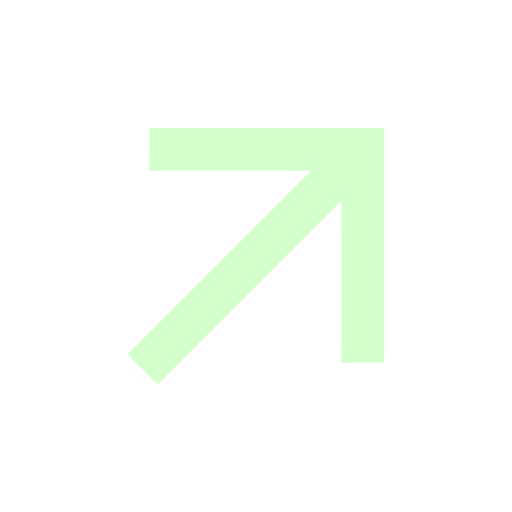
Why does Anymal use blockchain?
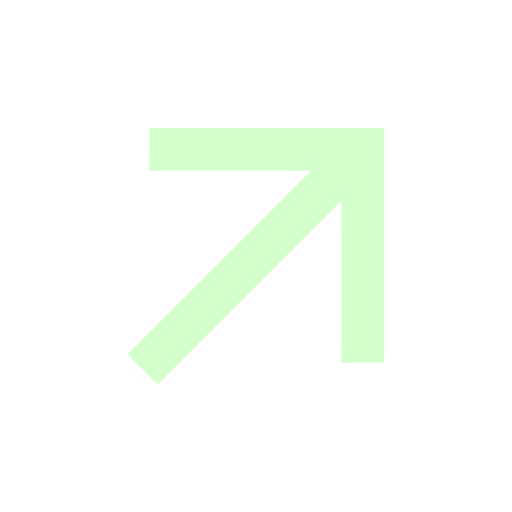
What composable primitives does Anymal provide?
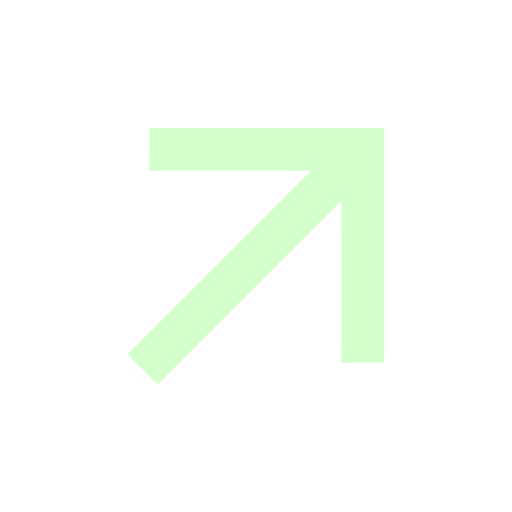
What is the Universal Animal ID, and why is it important?
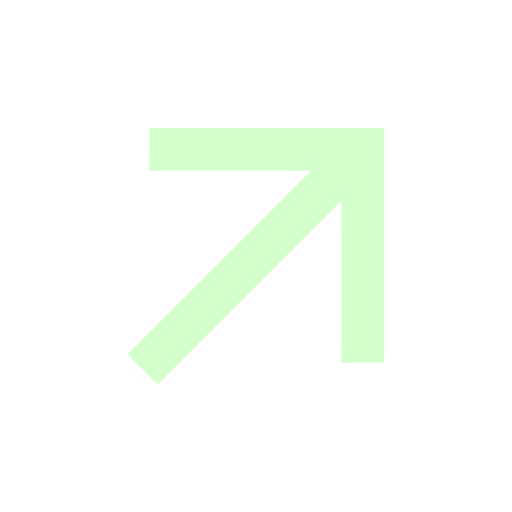
What are the benefits of using Anymal Protocol?
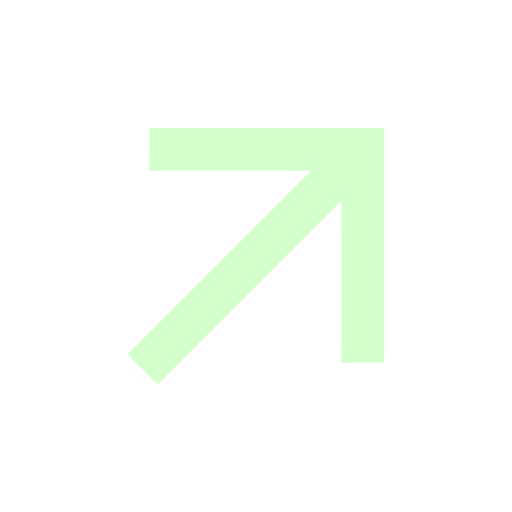
What are $KBL tokens, and how are they used?
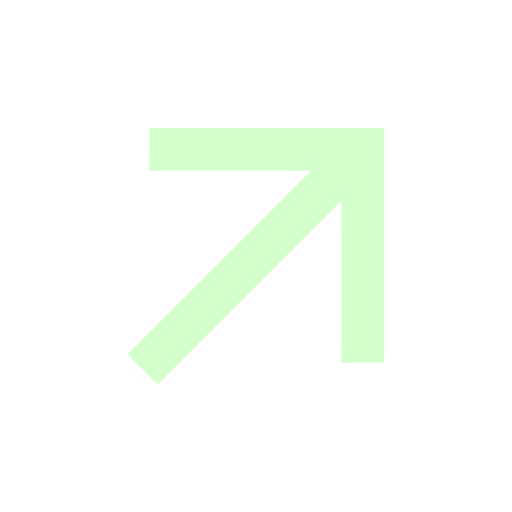
What is Anymal Protocol?
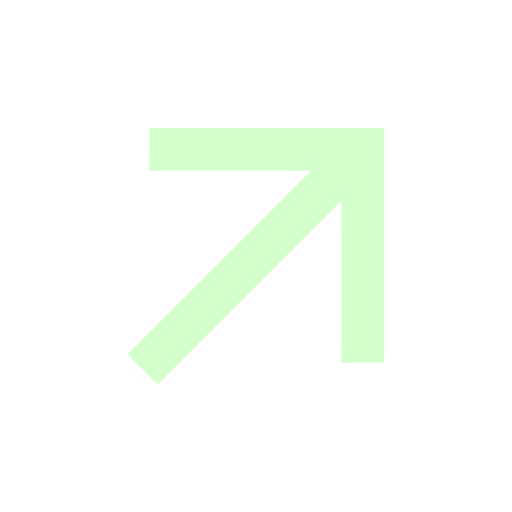
Why does Anymal use blockchain?
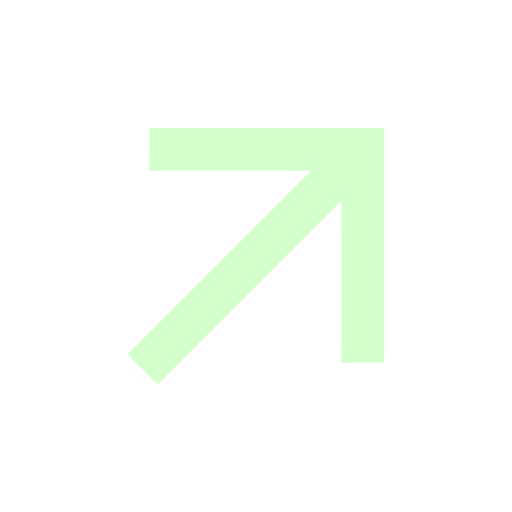
What composable primitives does Anymal provide?
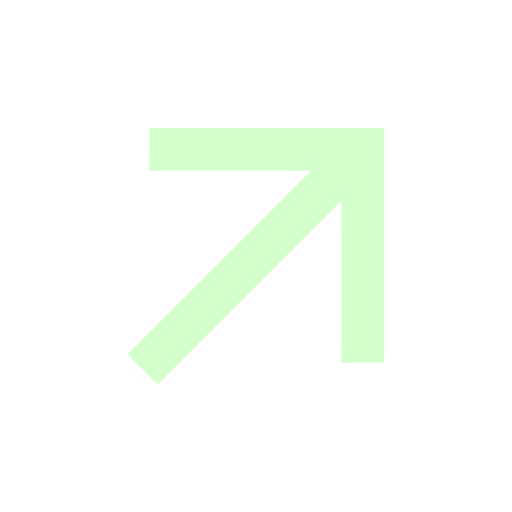
What is the Universal Animal ID, and why is it important?
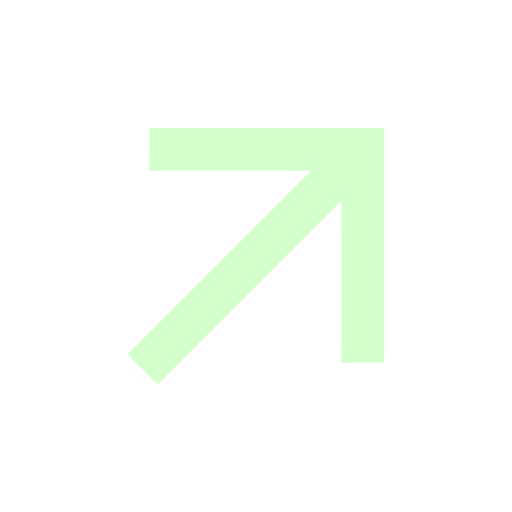
What are the benefits of using Anymal Protocol?
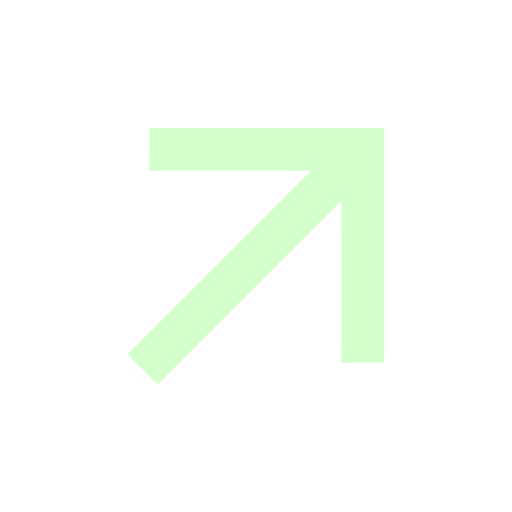
What are $KBL tokens, and how are they used?
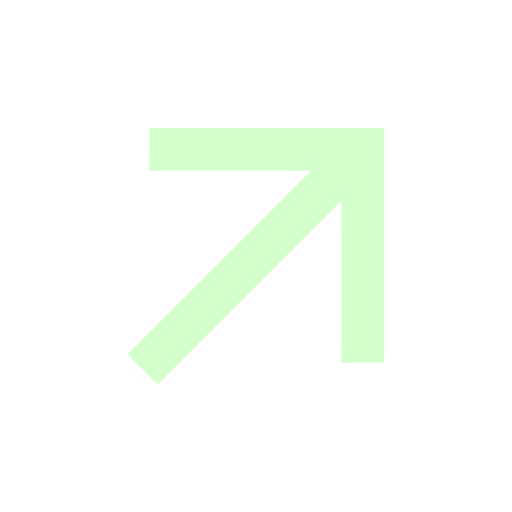
What is Anymal Protocol?
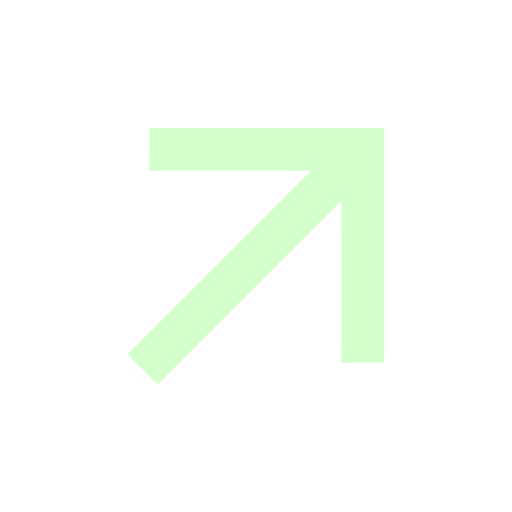
Why does Anymal use blockchain?
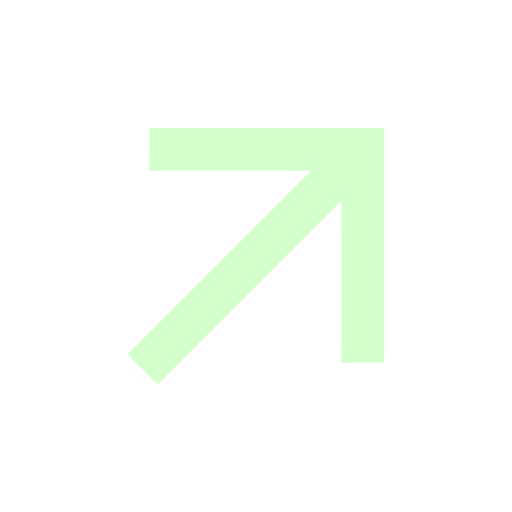
What composable primitives does Anymal provide?
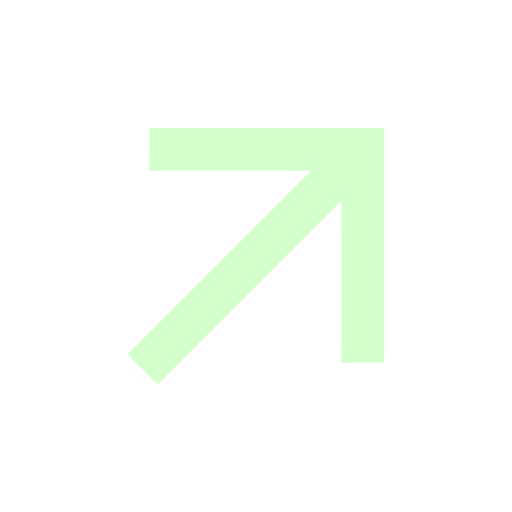
What is the Universal Animal ID, and why is it important?
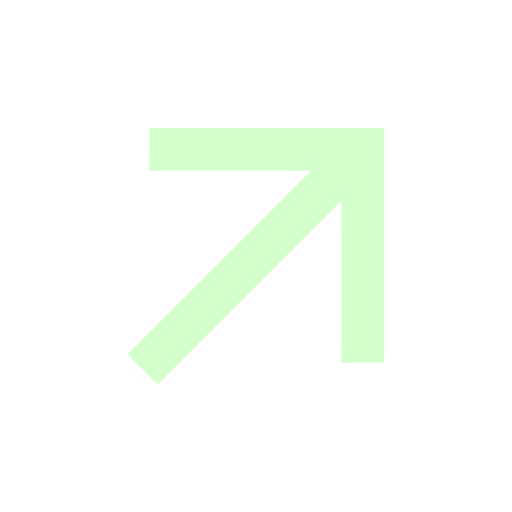
What are the benefits of using Anymal Protocol?
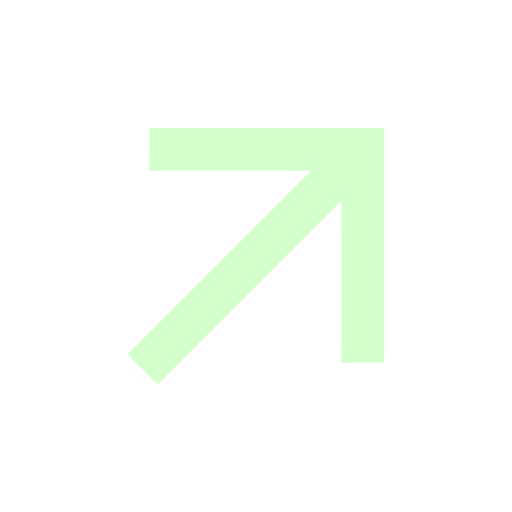
What are $KBL tokens, and how are they used?
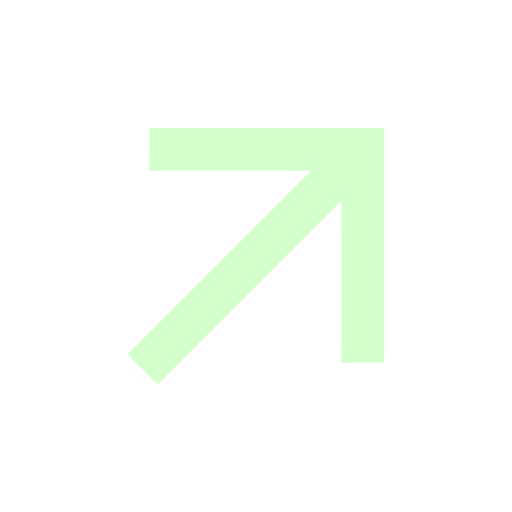
What is Anymal Protocol?
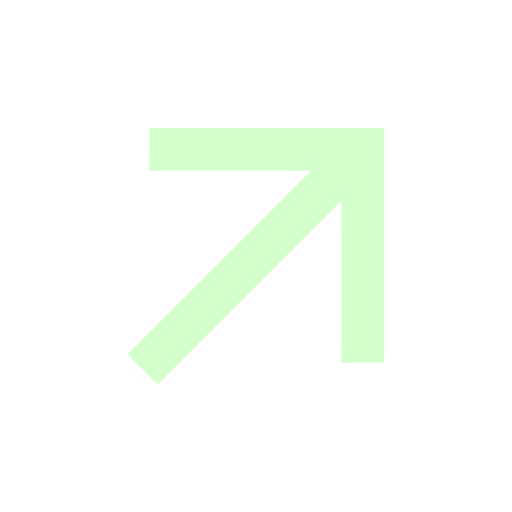
Why does Anymal use blockchain?
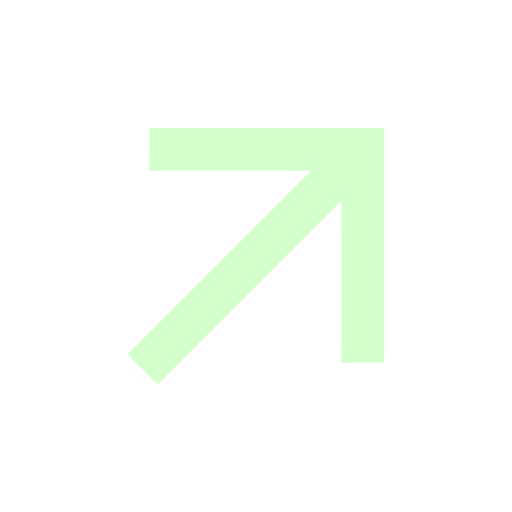
What composable primitives does Anymal provide?
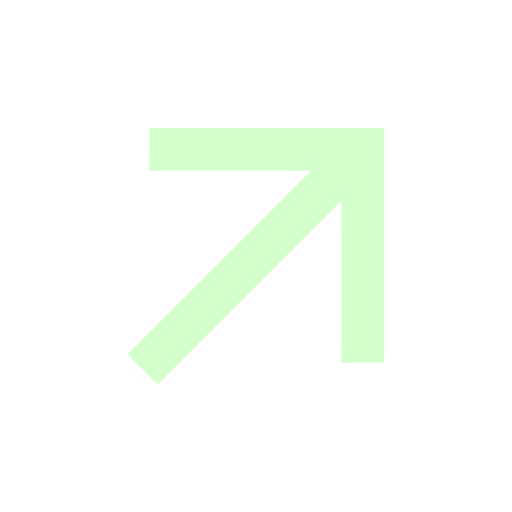
What is the Universal Animal ID, and why is it important?
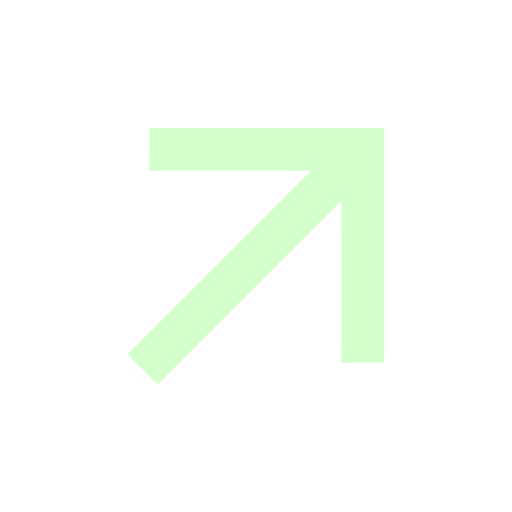
What are the benefits of using Anymal Protocol?
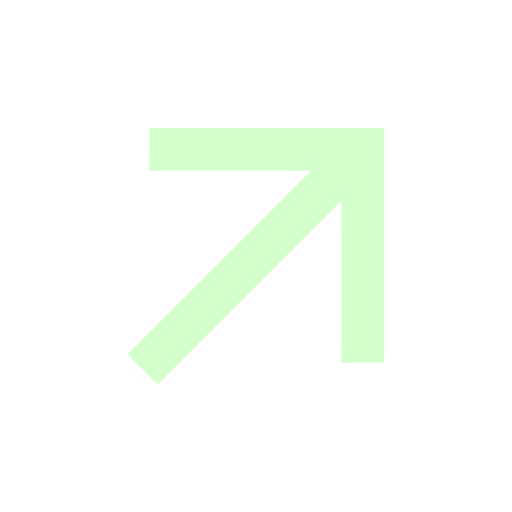
What are $KBL tokens, and how are they used?
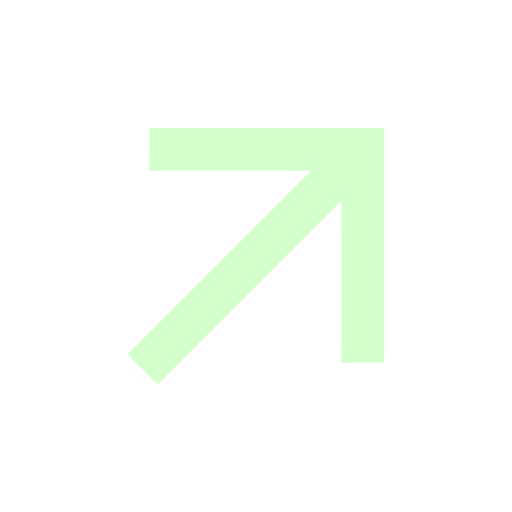
What is Anymal Protocol?
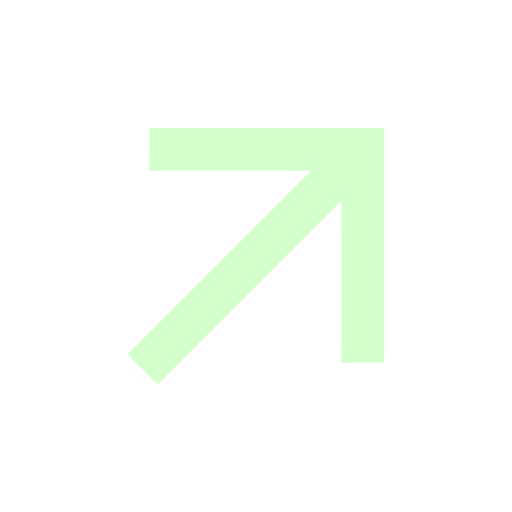
Why does Anymal use blockchain?
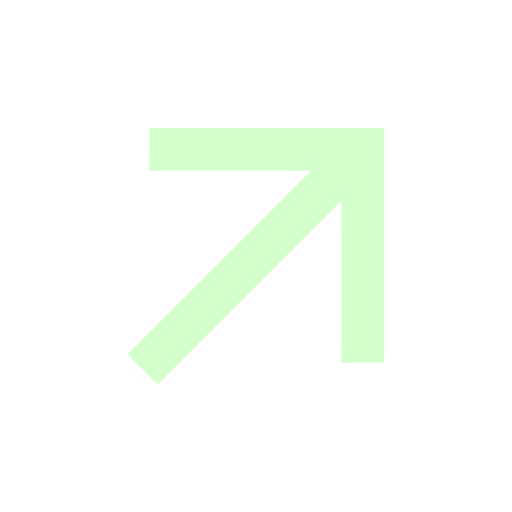
What composable primitives does Anymal provide?
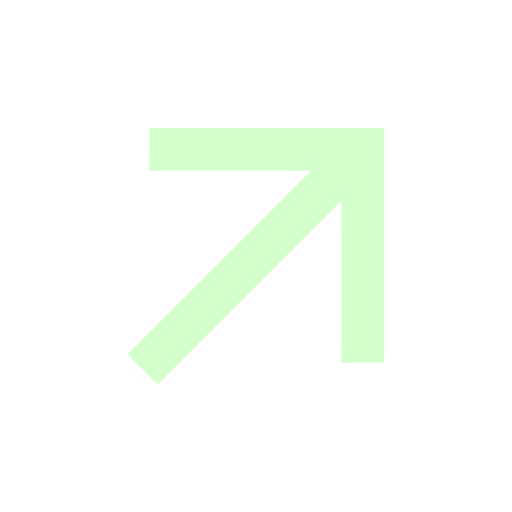
What is the Universal Animal ID, and why is it important?
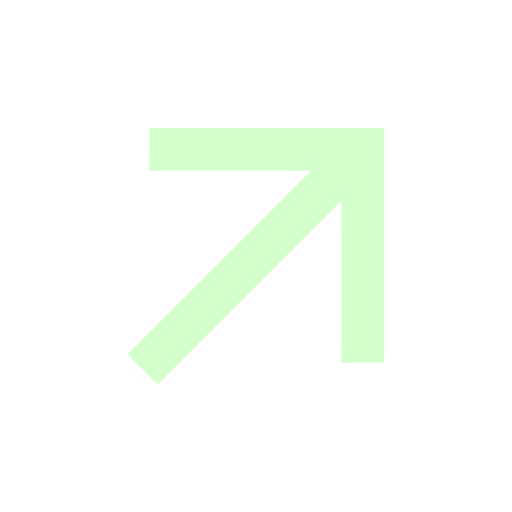
What are the benefits of using Anymal Protocol?
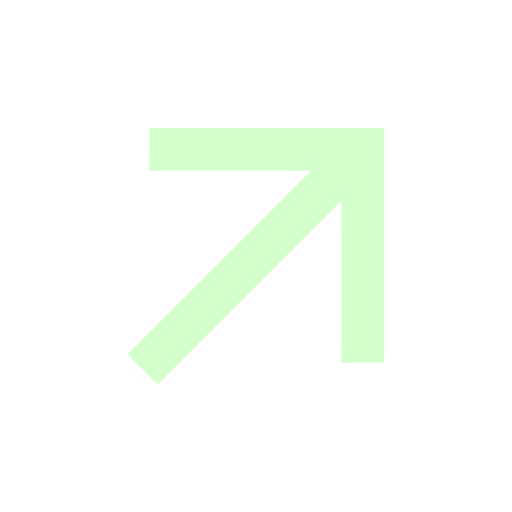
What are $KBL tokens, and how are they used?